Tutorial for NIRCam coronagraphy reduction with spaceKLIP
In this notebook we will reduce the NIRCam coronagraphy data on HIP 65426 b from the JWST ERS program on Direct Observations of Exoplanetary Systems, program 1386.
Relation to other tutorials: This notebook is intentionally very similar to the MIRI data reduction notebook. Subsequent analyses steps will be carried out in the NIRCam post-pipeline analyses notebook.
NIRCam-specific information: Steps and information specific to NIRCam are called out in blue.
Table of Contents:
Setup and imports
[1]:
import os
import pdb
import sys
import glob
import numpy as np
import astropy.io.fits as fits
import matplotlib.pyplot as plt
import matplotlib
matplotlib.rcParams.update({'font.size': 14})
import spaceKLIP
Note that currently the import of webbpsf_ext
has a side effect of configuring extra verbose logging. We’re not interested in that logging text, so let’s quiet it.
[2]:
import webbpsf_ext
webbpsf_ext.setup_logging('WARN', verbose=False)
Precursor: Download the data, if you don’t already have it.
If you already have a copy of this data, then you can adjust paths below. For this notebook, we assume you don’t already have the data. So let’s download it here.
We can do this using the (jwst_mast_query)[https://github.com/spacetelescope/jwst_mast_query] package. Consult that package’s own documentation for more.
We download all the uncalibrated raw data (uncal.fits
), since we will use spaceKLIP to invoke the jwst pipeline with some customized options and extra steps optimized for coronagraphy.
[4]:
# name the subdirectory where we will keep the data for this tutorial
data_root = 'data_nircam_hd65426'
[5]:
# make subdirectories to put these data in
if not os.path.isdir(data_root):
os.makedirs(data_root)
os.makedirs(os.path.join(data_root, 'uncal'))
# invoke the download
download_cmd = "jwst_download.py --propID 1386 -i nircam -l 700 --obsnums 1 2 3 "+\
"--outsubdir data_nircam_hd65426/uncal --skip_propID2outsubdir -f uncal"
import subprocess
subprocess.Popen(download_cmd, shell=True).wait()
Level 1 reductions
Index files into database for level 1
SpaceKLIP relies on a Database
class to track observations, data files, and the relationships between them.
We begin by creating a database, and reading files into it.
For purposes of this tutorial, let’s only reduce one filter’s worth of data.
[6]:
filt = 'F444W' # set this to None to disable filter selection when loading data in, and load all filters
[7]:
# Initialize spaceKLIP database.
database = spaceKLIP.database.create_database(input_dir = os.path.join(data_root, 'uncal'),
output_dir=data_root,
filt=filt,
pid=1386)
[spaceKLIP.database:INFO] --> Identified 1 concatenation(s)
[spaceKLIP.database:INFO] --> Concatenation 1: JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- --------------- ... ----------------- ----------- -------- ------- ------- ---------- ----- ------------------ --------
SCI NRC_CORON STAGE0 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN 110.2786558985023 nan
SCI NRC_CORON STAGE0 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN 120.3590194808035 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.00836165914811 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0083913507891 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0084136911584 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.00840409160931 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0083955337505 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0083853392871 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0083644991754 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.0083913349947 nan
REF NRC_CORON STAGE0 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN 110.00840088933231 nan
The above is a bit verbose and can be hard for a human to parse; let’s ask the database to summarize what it contains:
[8]:
database.summarize()
NIRCAM_F444W_MASK335R
STAGE0: 11 files; 2 SCI, 9 REF
Run level 1 reductions
We use spaceKLIP to invoke the level 1 pipeline, with a handful of parameter customizations.
This will run the Detector1 pipeline for all input data, saving the output to a subdirectory named stage1
.
[9]:
spaceKLIP.coron1pipeline.run_obs(database=database,
steps={'saturation': {'n_pix_grow_sat': 1,
'grow_diagonal': False},
'refpix': {'odd_even_columns': True,
'odd_even_rows': True,
'nlower': 4,
'nupper': 4,
'nleft': 4,
'nright': 4,
'nrow_off': 0,
'ncol_off': 0},
'dark_current': {'skip': True},
'persistence': {'skip': True},
'jump': {'rejection_threshold': 4.,
'three_group_rejection_threshold': 4.,
'four_group_rejection_threshold': 4.,
'maximum_cores': 'all'},
'ramp_fit': {'save_calibrated_ramp': False,
'maximum_cores': 'all'}},
subdir='stage1')
[spaceKLIP.coron1pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386002001_0310a_00001_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386003001_0310a_00001_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00001_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00002_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00003_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00004_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00005_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00006_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00007_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00008_nrcalong_uncal.fits
[spaceKLIP.coron1pipeline:INFO] --> Coron1Pipeline: processing jw01386001001_0310e_00009_nrcalong_uncal.fits
We can now examine the database, and it shows that all the available files for both filters have now been processed to Stage 1.
(The stage 0 files are removed from the database automatically since there is nothing more to do with them, though the files remain on disk of course)
[10]:
database.summarize()
NIRCAM_F444W_MASK335R
STAGE1: 11 files; 2 SCI, 9 REF
Display the results of the level 1 reductions
For example, let’s look at the data available in one filter, F444W. Each image is displayed with some annotations. The pixels masked out as DO_NOT_USE in the data quality extension are masked out in orange.
[11]:
spaceKLIP.plotting.display_coron_dataset(database,
restrict_to='F444W', save_filename='plots_f444w_stage1.pdf')
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T05:06:00.117' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T05:11:09.069' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T05:16:18.022' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.360184 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.180767 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706122045.054 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T00:56:04.604' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T00:56:46.296' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T00:57:27.989' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.407974 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.232067 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706579072.560 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T00:57:58.524' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T00:58:40.216' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T00:59:21.909' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.407619 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.231686 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706575662.382 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T00:59:51.356' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:00:33.048' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:01:14.741' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.407268 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.231308 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706572284.761 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:01:44.188' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:02:25.880' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:03:07.573' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.406917 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.230931 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706568907.128 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:03:39.196' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:04:20.888' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:05:02.581' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.406558 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.230546 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706565464.343 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:05:30.940' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:06:12.632' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:06:54.325' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.406211 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.230172 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706562119.254 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:07:23.708' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:08:05.400' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:08:47.093' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.405859 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.229794 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706558743.499 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:09:17.627' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:09:59.319' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:10:41.012' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.405505 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.229413 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706555333.275 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:11:10.459' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T01:11:52.151' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T01:12:33.844' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.405153 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.229035 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706551955.574 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]











Level 2 reductions
Optional: Re-read level 1 outputs into database
This shows how you can start re-reductions at this point, once you have run the previous steps.
[12]:
database = spaceKLIP.database.create_database(input_dir = os.path.join(data_root, 'stage1'),
file_type='rateints.fits',
output_dir=data_root,
pid=1386)
[spaceKLIP.database:INFO] --> Identified 5 concatenation(s)
[spaceKLIP.database:INFO] --> Concatenation 1: JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ----- ------------------ --------
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 110.2786614838192 nan
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 120.3590367370958 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084122158856 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083878498123 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.00838992902911 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.008379705443 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083798110777 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083885799926 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083749081301 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083907725108 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083938406817 nan
[spaceKLIP.database:INFO] --> Concatenation 2: JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ ------------------ ... ----------------- ----------- -------- ------- ------- ---------- ----- ----------------- --------
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 110.2786597837088 nan
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 120.3590291363088 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084057157205 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083749896574 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083976791623 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083837712263 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083853736149 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083784141108 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083730040159 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084000779611 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083892155217 nan
[spaceKLIP.database:INFO] --> Concatenation 3: JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ ------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ----- ------------------ --------
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 110.2786565966635 nan
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 120.3590218755254 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083775922736 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083738778466 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084043651638 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083604696634 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083894112827 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.008387378849 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.00838756252931 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084015860713 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083774942899 nan
[spaceKLIP.database:INFO] --> Concatenation 4: JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ----- ----------------- --------
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 110.278658665248 nan
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 120.3590255905366 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083830790994 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083958757078 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084069391043 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083636119141 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083844523855 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084027568964 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083947382326 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083666014151 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084090086911 nan
[spaceKLIP.database:INFO] --> Concatenation 5: JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- --------------- ... ----------------- ----------- -------- ------- ------- ---------- ----- ------------------ --------
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 110.2786558985023 nan
SCI NRC_CORON STAGE1 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 DN/s 120.3590194808035 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.00836165914811 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083913507891 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0084136911584 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.00840409160931 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083955337505 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083853392871 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083644991754 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.0083913349947 nan
REF NRC_CORON STAGE1 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 DN/s 110.00840088933231 nan
Run level 2 pipeline
Now we run the stage 2 pipeline. Little customization is needed here.
[13]:
spaceKLIP.coron2pipeline.run_obs(database=database,
steps={'outlier_detection': {'skip': False}},
)
[spaceKLIP.coron2pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386002001_03106_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386003001_03106_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00002_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00003_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00004_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00005_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00006_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00007_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00008_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03106_00009_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386002001_03107_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386003001_03107_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00002_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00003_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00004_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00005_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00006_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00007_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00008_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_03108_00009_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386002001_03109_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386003001_03109_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00002_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00003_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00004_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00005_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00006_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00007_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00008_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310c_00009_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386002001_03108_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386003001_03108_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00002_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00003_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00004_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00005_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00006_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00007_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00008_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310a_00009_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386002001_0310a_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386003001_0310a_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00001_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00002_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00003_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00004_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00005_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00006_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00007_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00008_nrcalong_rateints.fits
[spaceKLIP.coron2pipeline:INFO] --> Coron2Pipeline: processing jw01386001001_0310e_00009_nrcalong_rateints.fits
And again, we can check the database now contains level 2 reduces versions of all the files:
[14]:
database.summarize()
NIRCAM_F250M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
NIRCAM_F300M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
NIRCAM_F356W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
NIRCAM_F410M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
NIRCAM_F444W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
Display the results of the level 2 reductions
These look nearly identical to the stage 1 outputs, but note the image display units have been rescaled from DN/s into physical units of MJy/sr.
[15]:
spaceKLIP.plotting.display_coron_dataset(database,
restrict_to='F444W', save_filename='plots_f444w_stage2.pdf')
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]











Level 3 reductions: Preparations
Preparations for PSF subtraction:
As is often the case in high contrast imaging, getting good PSF subtractions depends sensitively on preparing the data ahead of time.
Here we take special care about image centering, background subtractions, and bad pixel replacement/interpolation, all prior to the PSF subtraction steps
Optional: Re-read level 2 outputs into database
This shows how you can start re-reductions at this point, once you have run the previous steps.
[16]:
database = spaceKLIP.database.create_database(input_dir = os.path.join(data_root, 'stage2'),
file_type='calints.fits',
output_dir=data_root,
pid=1386)
[spaceKLIP.database:INFO] --> Identified 5 concatenation(s)
[spaceKLIP.database:INFO] --> Concatenation 1: JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ------ ------------------ --------
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 110.2786614838192 nan
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 120.3590367370958 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084122158856 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083878498123 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.00838992902911 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.008379705443 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083798110777 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083885799926 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083749081301 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083907725108 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083938406817 nan
[spaceKLIP.database:INFO] --> Concatenation 2: JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ ------------------ ... ----------------- ----------- -------- ------- ------- ---------- ------ ----------------- --------
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 110.2786597837088 nan
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 120.3590291363088 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084057157205 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083749896574 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083976791623 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083837712263 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083853736149 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083784141108 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083730040159 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084000779611 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F300M 2.9940444469417997 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083892155217 nan
[spaceKLIP.database:INFO] --> Concatenation 3: JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ ------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ------ ------------------ --------
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 110.2786565966635 nan
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 120.3590218755254 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083775922736 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083738778466 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084043651638 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083604696634 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083894112827 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.008387378849 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.00838756252931 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084015860713 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083774942899 nan
[spaceKLIP.database:INFO] --> Concatenation 4: JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----------------- ----------- -------- ------- ------- ---------- ------ ----------------- --------
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 110.278658665248 nan
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 120.3590255905366 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083830790994 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083958757078 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084069391043 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083636119141 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083844523855 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084027568964 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083947382326 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083666014151 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084090086911 nan
[spaceKLIP.database:INFO] --> Concatenation 5: JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... EFFINTTM SUBARRAY NUMDTHPT XOFFSET YOFFSET PIXSCALE BUNIT ROLL_REF BLURFWHM
---- --------- -------- -------- --------- -------- -------- ------ --------------- --------------- ... ----------------- ----------- -------- ------- ------- ---------- ------ ------------------ --------
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 110.2786558985023 nan
SCI NRC_CORON STAGE2 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 307.88352 SUB320A335R 1 0.0 0.0 0.06247899 MJy/sr 120.3590194808035 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.00836165914811 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083913507891 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0084136911584 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.00840409160931 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083955337505 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083853392871 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083644991754 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.0083913349947 nan
REF NRC_CORON STAGE2 JWST HIP-68245 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 40.62352000000001 SUB320A335R 9 0.0 0.0 0.06247899 MJy/sr 110.00840088933231 nan
Extra image processing to improve coronagraphic reductions
[17]:
# Initialize spaceKLIP image manipulation tools class.
imageTools = spaceKLIP.imagetools.ImageTools(database=database)
NIRCam-specific information: The subtract_median background subtraction step is only recommended for NIRCam, not MIRI.
[18]:
# Median-subtract each frame to mitigate uncalibrated bias drifts.
imageTools.subtract_median(types=['SCI', 'SCI_TA', 'SCI_BG', 'REF', 'REF_TA', 'REF_BG'],
subdir='medsub')
[spaceKLIP.imagetools:INFO] Median subtraction using method=border
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386002001_03106_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.09
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386003001_03106_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.09
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.15
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00002_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.17
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00003_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.13
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00004_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.22
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00005_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.09
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00006_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.08
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00007_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.13
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00008_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.37
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03106_00009_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.12
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386002001_03107_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.07
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386003001_03107_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.07
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.15
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00002_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.02
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00003_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.03
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00004_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.00
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00005_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.04
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00006_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.08
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00007_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.03
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00008_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.13
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_03108_00009_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.12
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386002001_03109_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.03
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386003001_03109_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.04
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = -0.05
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00002_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = -0.05
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00003_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = -0.07
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00004_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.01
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00005_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.01
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00006_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.06
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00007_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.03
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00008_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.03
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310c_00009_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.02
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386002001_03108_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.06
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386003001_03108_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.07
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.14
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00002_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.11
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00003_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.01
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00004_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.11
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00005_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.05
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00006_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.12
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00007_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.02
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00008_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.16
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310a_00009_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.12
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386002001_0310a_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.08
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386003001_0310a_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.09
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00001_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.16
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00002_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.11
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00003_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.13
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00004_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.15
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00005_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.08
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00006_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.09
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00007_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.13
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00008_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.15
[spaceKLIP.imagetools:INFO] --> Median subtraction: jw01386001001_0310e_00009_nrcalong_calints.fits
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Median subtraction: mean of frame median = 0.10
For NIRCam, the JWST pipeline does not sufficiently repair bad pixels (i.e. anomalous outliers) within the coronagraphic subarrays.
Here we use custom functions within spaceKLIP to detect and repair bad pixels.
NIRCam-specific information: The fix_bad_pixels step was developed originally for NIRCam and is tuned to work pretty well on NIRCam data.
[19]:
# Fix bad pixels using custom spaceKLIP routines. Multiple routines can be
# combined in a custom order by joining them with a + sign.
# - bpclean: use sigma clipping to find additional bad pixels.
# - custom: use custom map to find additional bad pixels.
# - timemed: replace pixels which are only bad in some frames with their
# median value from the good frames.
# - dqmed: replace bad pixels with the median of surrounding good
# pixels.
# - medfilt: replace bad pixels with an image plane median filter.
imageTools.fix_bad_pixels(method='bpclean+timemed+dqmed+medfilt',
bpclean_kwargs={'sigclip': 3,
'shift_x': [-1, 0, 1],
'shift_y': [-1, 0, 1]},
custom_kwargs={},
timemed_kwargs={},
dqmed_kwargs={'shift_x': [-1, 0, 1],
'shift_y': [-1, 0, 1]},
medfilt_kwargs={'size': 4},
subdir='bpcleaned')
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386002001_03106_00001_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8684 additional bad pixel(s) -- 2.12%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8343 bad pixel(s) -- 2.04%
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/numpy/lib/nanfunctions.py:1217: RuntimeWarning: All-NaN slice encountered
[py.warnings:WARNING] return function_base._ureduce(a, func=_nanmedian, keepdims=keepdims,
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1800 bad pixel(s) -- 0.44%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386003001_03106_00001_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8295 additional bad pixel(s) -- 2.03%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 7964 bad pixel(s) -- 1.94%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1768 bad pixel(s) -- 0.43%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00001_nrcalong_calints.fits
Frame 1/4, iteration 1
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/numpy/lib/nanfunctions.py:1872: RuntimeWarning: Degrees of freedom <= 0 for slice.
[py.warnings:WARNING] var = nanvar(a, axis=axis, dtype=dtype, out=out, ddof=ddof,
[py.warnings:WARNING]
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9105 additional bad pixel(s) -- 2.22%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8913 bad pixel(s) -- 2.18%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1668 bad pixel(s) -- 0.41%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00002_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8938 additional bad pixel(s) -- 2.18%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8844 bad pixel(s) -- 2.16%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1636 bad pixel(s) -- 0.40%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00003_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9047 additional bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8790 bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1700 bad pixel(s) -- 0.42%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00004_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9429 additional bad pixel(s) -- 2.30%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 9200 bad pixel(s) -- 2.25%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1716 bad pixel(s) -- 0.42%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00005_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9053 additional bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8843 bad pixel(s) -- 2.16%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1716 bad pixel(s) -- 0.42%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00006_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9045 additional bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8764 bad pixel(s) -- 2.14%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1712 bad pixel(s) -- 0.42%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00007_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9151 additional bad pixel(s) -- 2.23%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8977 bad pixel(s) -- 2.19%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1664 bad pixel(s) -- 0.41%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00008_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8792 additional bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8554 bad pixel(s) -- 2.09%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1712 bad pixel(s) -- 0.42%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03106_00009_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9186 additional bad pixel(s) -- 2.24%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8976 bad pixel(s) -- 2.19%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1660 bad pixel(s) -- 0.41%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386002001_03107_00001_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8331 additional bad pixel(s) -- 2.03%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8189 bad pixel(s) -- 2.00%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1600 bad pixel(s) -- 0.39%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386003001_03107_00001_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8196 additional bad pixel(s) -- 2.00%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8075 bad pixel(s) -- 1.97%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1580 bad pixel(s) -- 0.39%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00001_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8809 additional bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8718 bad pixel(s) -- 2.13%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1532 bad pixel(s) -- 0.37%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00002_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9071 additional bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8947 bad pixel(s) -- 2.18%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1540 bad pixel(s) -- 0.38%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00003_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8984 additional bad pixel(s) -- 2.19%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8848 bad pixel(s) -- 2.16%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1564 bad pixel(s) -- 0.38%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00004_nrcalong_calints.fits
Frame 4/4, iteration 7
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9136 additional bad pixel(s) -- 2.23%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 9064 bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1552 bad pixel(s) -- 0.38%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00005_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8934 additional bad pixel(s) -- 2.18%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8899 bad pixel(s) -- 2.17%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1568 bad pixel(s) -- 0.38%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00006_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 8909 additional bad pixel(s) -- 2.18%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8800 bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1532 bad pixel(s) -- 0.37%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00007_nrcalong_calints.fits
Frame 4/4, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9003 additional bad pixel(s) -- 2.20%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 8861 bad pixel(s) -- 2.16%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1600 bad pixel(s) -- 0.39%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00008_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9231 additional bad pixel(s) -- 2.25%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 9070 bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1600 bad pixel(s) -- 0.39%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_03108_00009_nrcalong_calints.fits
Frame 4/4, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 9102 additional bad pixel(s) -- 2.22%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 9032 bad pixel(s) -- 2.21%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1552 bad pixel(s) -- 0.38%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386002001_03109_00001_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3546 additional bad pixel(s) -- 1.73%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3279 bad pixel(s) -- 1.60%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 996 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 16 bad pixel(s) -- 0.01%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386003001_03109_00001_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3652 additional bad pixel(s) -- 1.78%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3376 bad pixel(s) -- 1.65%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1006 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 4 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00001_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4064 additional bad pixel(s) -- 1.98%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3817 bad pixel(s) -- 1.86%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1068 bad pixel(s) -- 0.52%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00002_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4000 additional bad pixel(s) -- 1.95%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3719 bad pixel(s) -- 1.82%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1036 bad pixel(s) -- 0.51%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00003_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3971 additional bad pixel(s) -- 1.94%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3736 bad pixel(s) -- 1.82%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1014 bad pixel(s) -- 0.50%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00004_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4075 additional bad pixel(s) -- 1.99%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3849 bad pixel(s) -- 1.88%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1008 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00005_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3950 additional bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3716 bad pixel(s) -- 1.81%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 992 bad pixel(s) -- 0.48%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00006_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3950 additional bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3714 bad pixel(s) -- 1.81%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1002 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00007_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3947 additional bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3752 bad pixel(s) -- 1.83%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1050 bad pixel(s) -- 0.51%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 2 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00008_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3959 additional bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3702 bad pixel(s) -- 1.81%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 998 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310c_00009_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4046 additional bad pixel(s) -- 1.98%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3780 bad pixel(s) -- 1.85%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1022 bad pixel(s) -- 0.50%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386002001_03108_00001_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3915 additional bad pixel(s) -- 1.91%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3630 bad pixel(s) -- 1.77%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 986 bad pixel(s) -- 0.48%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386003001_03108_00001_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3886 additional bad pixel(s) -- 1.90%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3570 bad pixel(s) -- 1.74%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1006 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00001_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4408 additional bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4126 bad pixel(s) -- 2.01%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1070 bad pixel(s) -- 0.52%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00002_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4426 additional bad pixel(s) -- 2.16%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4138 bad pixel(s) -- 2.02%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1100 bad pixel(s) -- 0.54%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00003_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4229 additional bad pixel(s) -- 2.06%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3949 bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1068 bad pixel(s) -- 0.52%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00004_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4291 additional bad pixel(s) -- 2.10%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4003 bad pixel(s) -- 1.95%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1086 bad pixel(s) -- 0.53%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00005_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4293 additional bad pixel(s) -- 2.10%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4021 bad pixel(s) -- 1.96%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1070 bad pixel(s) -- 0.52%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00006_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4407 additional bad pixel(s) -- 2.15%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4115 bad pixel(s) -- 2.01%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1392 bad pixel(s) -- 0.68%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 2 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00007_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4348 additional bad pixel(s) -- 2.12%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4074 bad pixel(s) -- 1.99%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1108 bad pixel(s) -- 0.54%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00008_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4333 additional bad pixel(s) -- 2.12%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4107 bad pixel(s) -- 2.01%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1022 bad pixel(s) -- 0.50%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310a_00009_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4480 additional bad pixel(s) -- 2.19%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 4197 bad pixel(s) -- 2.05%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1012 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386002001_0310a_00001_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4498 additional bad pixel(s) -- 2.20%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3838 bad pixel(s) -- 1.87%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1362 bad pixel(s) -- 0.67%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386003001_0310a_00001_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4694 additional bad pixel(s) -- 2.29%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3881 bad pixel(s) -- 1.90%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1492 bad pixel(s) -- 0.73%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 2 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00001_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4026 additional bad pixel(s) -- 1.97%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3801 bad pixel(s) -- 1.86%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1026 bad pixel(s) -- 0.50%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00002_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4007 additional bad pixel(s) -- 1.96%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3767 bad pixel(s) -- 1.84%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 964 bad pixel(s) -- 0.47%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00003_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4080 additional bad pixel(s) -- 1.99%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3871 bad pixel(s) -- 1.89%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 982 bad pixel(s) -- 0.48%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00004_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4023 additional bad pixel(s) -- 1.96%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3807 bad pixel(s) -- 1.86%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1010 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00005_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3995 additional bad pixel(s) -- 1.95%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3757 bad pixel(s) -- 1.83%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1036 bad pixel(s) -- 0.51%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00006_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4091 additional bad pixel(s) -- 2.00%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3871 bad pixel(s) -- 1.89%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1008 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00007_nrcalong_calints.fits
Frame 2/2, iteration 5
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4017 additional bad pixel(s) -- 1.96%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3764 bad pixel(s) -- 1.84%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1076 bad pixel(s) -- 0.53%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00008_nrcalong_calints.fits
Frame 2/2, iteration 4
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 4067 additional bad pixel(s) -- 1.99%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3824 bad pixel(s) -- 1.87%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 1010 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
[spaceKLIP.imagetools:INFO] --> Method bpclean: jw01386001001_0310e_00009_nrcalong_calints.fits
Frame 2/2, iteration 6
[spaceKLIP.imagetools:INFO] --> Method bpclean: identified 3950 additional bad pixel(s) -- 1.93%
[spaceKLIP.imagetools:INFO] --> Method timemed: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method timemed: fixing 3728 bad pixel(s) -- 1.82%
[spaceKLIP.imagetools:INFO] --> Method dqmed: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method dqmed: fixing 998 bad pixel(s) -- 0.49%
[spaceKLIP.imagetools:INFO] --> Method medfilt: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Method medfilt: fixing 0 bad pixel(s) -- 0.00%
Finish pixel cleanup
Optinally, any remaining bad pixels we interpolate to replace NaNs with zero.
In this case, this step is not actually needed since already all the bad pixels have been fixed in the above step. So we can run this for example purposes, but it does not change any pixel values.
[20]:
# Replace nans.
imageTools.replace_nans(cval=0.,
types=['SCI', 'SCI_BG', 'REF', 'REF_BG'],
subdir='nanreplaced')
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
[spaceKLIP.imagetools:INFO] --> Nan replacement: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Nan replacement: replaced 0 nan pixel(s) with value 0.0 -- 0.00%
Improve PSF centering and alignment
NIRCam-specific information: These steps for update_center, recenter_frames, align_frames are only recommended for use on NIRCam data at this time.
Update NIRCam PSF center metadata, then recenter frames
This is an extra step to update header metadata for locations of the coronagraphs.
This uses a table of better center locations measured by Jarron Leisenring. Replaces the header values for the CRPIX locations for the mask locations.
Eventually the more precise location inormation will be in CRDS and this step will not be necessary
[21]:
# this changes only the mask center information in the database table. No change in the observed data
imageTools.update_nircam_centers()
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.26, 172.56)
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.29, 174.07)
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.98, 174.44)
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.41, 174.47)
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Update NIRCam coronagraphy centers: old = (150.10, 174.20), new = (150.38, 174.39)
To better measure the location of the star with respect to the coronagraph, we create a simulation of the star behind the coronagraph (using webbpsf), and cross-correlate this with the observed PSF. The cross correlation peak is used to infer the offset of the star relative to the mask center. The measured offset is used to shift the first frame to be centered. Then subsequent frames are aligned to that first frame.
The accuracy of this algorithm is around 7 milliarcsec according to testing.
This step also shifts to account for the coronagraph not being precisely centered in the subarray. After this step, the star center will be at the center of the pixel array.
[22]:
imageTools.recenter_frames(spectral_type='A2V')
# The spectral type is used to make a more accurate PSF simulation for the star
# This step changes the pixel data in the first science frame to center it.
# The other subsequent frames are shifted identically. I.e. the shift to center the first SCI frame
# is applied to all SCI and REF frames.
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: generating WebbPSF image for absolute centering (this might take a while)
[spaceKLIP.psf:INFO] Generating on-axis and off-axis PSFs...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/poppy/poppy/optics.py:464: DeprecationWarning: JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to webbpsf.NIRCam_BandLimitedCoron. The "nircamwedge" and "nircamcircular" options in poppy will be removed in a future version of poppy.
[py.warnings:WARNING] warnings.warn('JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to ' +
[py.warnings:WARNING]
[spaceKLIP.psf:INFO] Done.
[spaceKLIP.imagetools:INFO] --> Recenter frames: star offset from coronagraph center (dx, dy) = (0.18, -0.17) pix
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 970.51 mas
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: generating WebbPSF image for absolute centering (this might take a while)
[spaceKLIP.psf:INFO] Generating on-axis and off-axis PSFs...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/poppy/poppy/optics.py:464: DeprecationWarning: JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to webbpsf.NIRCam_BandLimitedCoron. The "nircamwedge" and "nircamcircular" options in poppy will be removed in a future version of poppy.
[py.warnings:WARNING] warnings.warn('JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to ' +
[py.warnings:WARNING]
[spaceKLIP.psf:INFO] Done.
[spaceKLIP.imagetools:INFO] --> Recenter frames: star offset from coronagraph center (dx, dy) = (0.18, -0.20) pix
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/matplotlib/transforms.py:209: ResourceWarning: unclosed file <_io.BufferedReader name='/Users/mperrin/software/webbpsf-data/MAST_JWST_WSS_OPDs/O2022073001-NRCA3_FP1-1.fits'>
[py.warnings:WARNING] self, lambda _, pop=child._parents.pop, k=id(self): pop(k))
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1039.60 mas
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: generating WebbPSF image for absolute centering (this might take a while)
[spaceKLIP.psf:INFO] Generating on-axis and off-axis PSFs...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/poppy/poppy/optics.py:464: DeprecationWarning: JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to webbpsf.NIRCam_BandLimitedCoron. The "nircamwedge" and "nircamcircular" options in poppy will be removed in a future version of poppy.
[py.warnings:WARNING] warnings.warn('JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to ' +
[py.warnings:WARNING]
[spaceKLIP.psf:INFO] Done.
[spaceKLIP.imagetools:INFO] --> Recenter frames: star offset from coronagraph center (dx, dy) = (0.14, -0.21) pix
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_recenter.pdf
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1031.63 mas
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: generating WebbPSF image for absolute centering (this might take a while)
[spaceKLIP.psf:INFO] Generating on-axis and off-axis PSFs...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/poppy/poppy/optics.py:464: DeprecationWarning: JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to webbpsf.NIRCam_BandLimitedCoron. The "nircamwedge" and "nircamcircular" options in poppy will be removed in a future version of poppy.
[py.warnings:WARNING] warnings.warn('JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to ' +
[py.warnings:WARNING]
[spaceKLIP.psf:INFO] Done.
[spaceKLIP.imagetools:INFO] --> Recenter frames: star offset from coronagraph center (dx, dy) = (0.13, -0.17) pix
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1057.41 mas
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: generating WebbPSF image for absolute centering (this might take a while)
[spaceKLIP.psf:INFO] Generating on-axis and off-axis PSFs...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/poppy/poppy/optics.py:464: DeprecationWarning: JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to webbpsf.NIRCam_BandLimitedCoron. The "nircamwedge" and "nircamcircular" options in poppy will be removed in a future version of poppy.
[py.warnings:WARNING] warnings.warn('JWST NIRCam specific functionality in poppy.BandLimitedCoron is moving to ' +
[py.warnings:WARNING]
[spaceKLIP.psf:INFO] Done.
[spaceKLIP.imagetools:INFO] --> Recenter frames: star offset from coronagraph center (dx, dy) = (0.13, -0.13) pix
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/matplotlib/cbook/__init__.py:2001: ResourceWarning: unclosed file <_io.BufferedReader name='/Users/mperrin/software/webbpsf-data/MAST_JWST_WSS_OPDs/O2022073001-NRCA3_FP1-1.fits'>
[py.warnings:WARNING] for attr in kwargs:
[py.warnings:WARNING]
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_recenter.pdf
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Recenter frames: median required shift = 1056.71 mas
This step saves output PDFs with plots showing the centering information results.
[38]:
!ls data_nircam_hd65426/recentered/*pdf
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_recenter.pdf
data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
[24]:
# Optional, open one of the plots to view it. (note, use of !open assumes you are running this on Mac OS)
!open data_nircam_hd65426/recentered/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASKA335R_SUB320A335R_recenter.pdf
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
Align Frames
This step applies shifts to the image pixel data to align frames. All subsequent frames are aligned to the first frame of the first science integration. (I.e. the second roll and all references are aligned to the first roll).
This measures and applies relative shifts between subsequent frames and the first frame.
[25]:
#Align Frames Use image registration to align all frames in a concatenation to the
# first science frame in that concatenation.
imageTools.align_frames(method='fourier',
kwargs={},
subdir='aligned')
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 0.08 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 5.94 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 12.81 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 33.38 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 24.92 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 11.13 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 2.55 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 10.47 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 24.61 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 34.09 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 37.00 mas
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 0.17 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 9.87 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 14.19 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 31.44 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 24.77 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.38 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 4.46 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.90 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 24.62 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 33.13 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 35.35 mas
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 0.15 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 9.81 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 14.15 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 31.93 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 24.58 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.31 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 5.79 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.87 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 26.51 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 32.61 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 35.59 mas
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 0.30 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 10.54 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 15.49 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 33.55 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 25.65 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.65 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 5.14 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 14.23 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 28.60 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 34.74 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 37.74 mas
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 0.32 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 13.10 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 16.96 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 36.76 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 29.19 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 15.39 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 3.42 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 14.24 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 28.58 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 35.42 mas
[spaceKLIP.imagetools:INFO] --> Align frames: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Align frames: median required shift = 39.75 mas
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
[spaceKLIP.imagetools:INFO] Plot saved in data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
[26]:
# This step also outputs plots that show the results of the alignment
!ls data_nircam_hd65426/aligned/*pdf
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASKA335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASKA335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASKA335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASKA335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R_align_sci.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASKA335R_SUB320A335R_align_sci.pdf
[27]:
# Open one to view the alignment of the reference images.
!open data_nircam_hd65426/aligned/JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASKA335R_SUB320A335R_align_ref.pdf
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
Pad empty space around frames
to give space to rotate and align during pyklip. THis puts a region of NAN pixels around the outside.
[28]:
# Pad all frames.
imageTools.pad_frames(npix=80,
cval=np.nan,
types=['SCI', 'SCI_BG', 'REF', 'REF_BG'],
subdir='padded')
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386002001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386003001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03106_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386002001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386003001_03107_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_03108_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386002001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386003001_03109_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310c_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386002001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386003001_03108_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310a_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386002001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386003001_0310a_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00001_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00002_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00003_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00004_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00005_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00006_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00007_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00008_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
[spaceKLIP.imagetools:INFO] --> Frame padding: jw01386001001_0310e_00009_nrcalong_calints.fits
[spaceKLIP.imagetools:INFO] --> Frame padding: old shape = (320, 320), new shape = (480, 480), fill value = nan
Display the cleaned datasets after all of the above
[29]:
spaceKLIP.plotting.display_coron_dataset(database,
restrict_to='F444W', save_filename='plots_f444w_stage2_cleaned.pdf')
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/enum.py:714: ResourceWarning: unclosed file <_io.BufferedReader name='/Users/mperrin/software/webbpsf-data/MAST_JWST_WSS_OPDs/O2022073001-NRCA3_FP1-1.fits'>
[py.warnings:WARNING] return cls.__new__(cls, value)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/plotting.py:212: RuntimeWarning: Mean of empty slice
[py.warnings:WARNING] image = np.nanmean(model.data, axis=0)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcsapi/fitswcs.py:346: UserWarning: 'WCS.all_world2pix' failed to converge to the requested accuracy.
[py.warnings:WARNING] After 20 iterations, the solution is diverging at least for one input point.
[py.warnings:WARNING] warnings.warn(str(e))
[py.warnings:WARNING]











[30]:
!open plots_f444w_stage2_cleaned.pdf
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
Level 3 reductions: KLIP
PSF Subtraction: option using pyKLIP
SpaceKLIP supports multiple algorithms for PSF subtraction, including pyKLIP (recommended) as well as the jwst pipeline Coron3Pipeline. Here we use a pyKLIP subtraction.
[31]:
# Run pyKLIP pipeline. Additional parameters for klip_dataset function can
# be passed using kwargs parameter.
spaceKLIP.pyklippipeline.run_obs(database=database,
kwargs={'mode': ['ADI', 'RDI', 'ADI+RDI'],
'annuli': [1, 5],
'subsections': [1],
'numbasis': [1, 2, 5, 10, 20, 50],
'algo': 'klip',
'save_rolls': True},
subdir='klipsub')
[spaceKLIP.pyklippipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.372585 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.194064 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706240147.863 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.505e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.368257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.189422 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706198895.066 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/pyklippipeline.py:159: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03106_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = fits.open(datapath)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 2.994e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 8, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.362558 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.183312 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706144631.810 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/pyklippipeline.py:159: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03107_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = fits.open(datapath)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 3.593e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.364924 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.185849 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706167152.529 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/pyklippipeline.py:159: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03109_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = fits.open(datapath)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.089e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.360184 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.180767 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706122045.054 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/pyklippipeline.py:159: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03108_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = fits.open(datapath)
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 1, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 1
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.pyklippipeline:INFO] --> pyKLIP: mode = ADI+RDI, annuli = 5, subsections = 1
Begin align and scale images for each wavelength
Wavelength 4.439e-06 with index 0 has finished align and scale. Queuing for KLIP
Total number of tasks for KLIP processing is 5
Closing threadpool
Derotating Images...
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
Writing Images to directory /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/docs/source/tutorials/data_nircam_hd65426/klipsub
wavelength collapsing reduced data of shape (b, N, wv, y, x):(6, 4, 1, 480, 480)
[spaceKLIP.database:INFO] --> Identified 5 concatenation(s)
[spaceKLIP.database:INFO] --> Concatenation 1: JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 2: JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ ------------------ ------------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 3: JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL PUPIL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ ------------- ---------------- ------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 4: JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 5: JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /var/folders/wj/p62fp78j0z1b4xyr2yql1prw00042w/T/ipykernel_27683/2016620805.py:3: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_0310a_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] spaceKLIP.pyklippipeline.run_obs(database=database,
[py.warnings:WARNING]
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- --------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
The stage 3 information in the database is added to another table. The stage 2 information remains in the database, which is needed to maintain the information on rolls and references used in the reduction for forward modeling. In fact the stage 3 outputs include a JSON file that includes the table of the stage 2 data, so if you read in the stage 3 outputs it also learns about the stage 2 inputs.
[32]:
database.summarize()
NIRCAM_F250M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F300M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F356W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F410M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F444W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
Optional: Re-read level 3 outputs into database
This shows how you can start re-analyses at this point, once you have run the previous steps.
Note, to read in level 3 data you must set the readlevel
parameter to 3. This invokes code for reading the level-3 formatted data products, and also implicitly reads in the metadata about the stage 2 files used as input to stage 3.
[33]:
database = spaceKLIP.database.create_database(input_dir = os.path.join(data_root, 'klipsub'),
file_type='*KLmodes-all.fits.fits',
output_dir=data_root,
readlevel=3,
pid=1386)
[spaceKLIP.database:INFO] --> Identified 5 concatenation(s)
[spaceKLIP.database:INFO] --> Concatenation 1: JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F250M 2.5049391569476 0.17829583911921 ... 8 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 2: JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ ------------------ ------------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F300M 2.9940444469417997 0.32555781627185004 ... 8 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 3: JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL PUPIL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ ------------- ---------------- ------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F356W 3.59344889657 0.72392951464142 MASKRND ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 4: JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- ---------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F410M 4.0886543870603 0.42628579023938 ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[spaceKLIP.database:INFO] --> Concatenation 5: JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
TYPE EXP_TYPE DATAMODL TELESCOP TARGPROP INSTRUME DETECTOR FILTER CWAVEL DWAVEL ... NINTS EFFINTTM SUBARRAY PIXSCALE MODE ANNULI SUBSECTS KLMODES BUNIT BLURFWHM
------ --------- -------- -------- --------- -------- -------- ------ --------------- --------------- ... ----- --------- ----------- ---------- ------- ------ -------- -------------- ------ --------
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI+RDI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 ADI 5 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 RDI 1 1 1,2,5,10,20,50 MJy/sr nan
PYKLIP NRC_CORON STAGE3 JWST HIP-65426 NIRCAM NRCALONG F444W 4.4393515120525 1.0676002928393 ... 4 307.88352 SUB320A335R 0.06247899 RDI 5 1 1,2,5,10,20,50 MJy/sr nan
[34]:
database.summarize()
NIRCAM_F250M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F300M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F356W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F410M_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
NIRCAM_F444W_MASK335R
STAGE2: 11 files; 2 SCI, 9 REF
STAGE3: 6 files; 6 PYKLIP
Note that both the L2 and L3 files are loaded automatically after calling read_jwst_s3_data
. This allows steps using or modeling the L3 data to have access to all the metadata about the individual exposures and orientations and PSFs that went into the L3 reductions.
[35]:
spaceKLIP.plotting.display_coron_dataset(database,
restrict_to='F444W', save_filename='plots_f444w_pyklip.pdf')
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'cdfix' made the change 'Success'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/stats/sigma_clipping.py:434: AstropyUserWarning: Input data contains invalid values (NaNs or infs), which were automatically clipped.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]





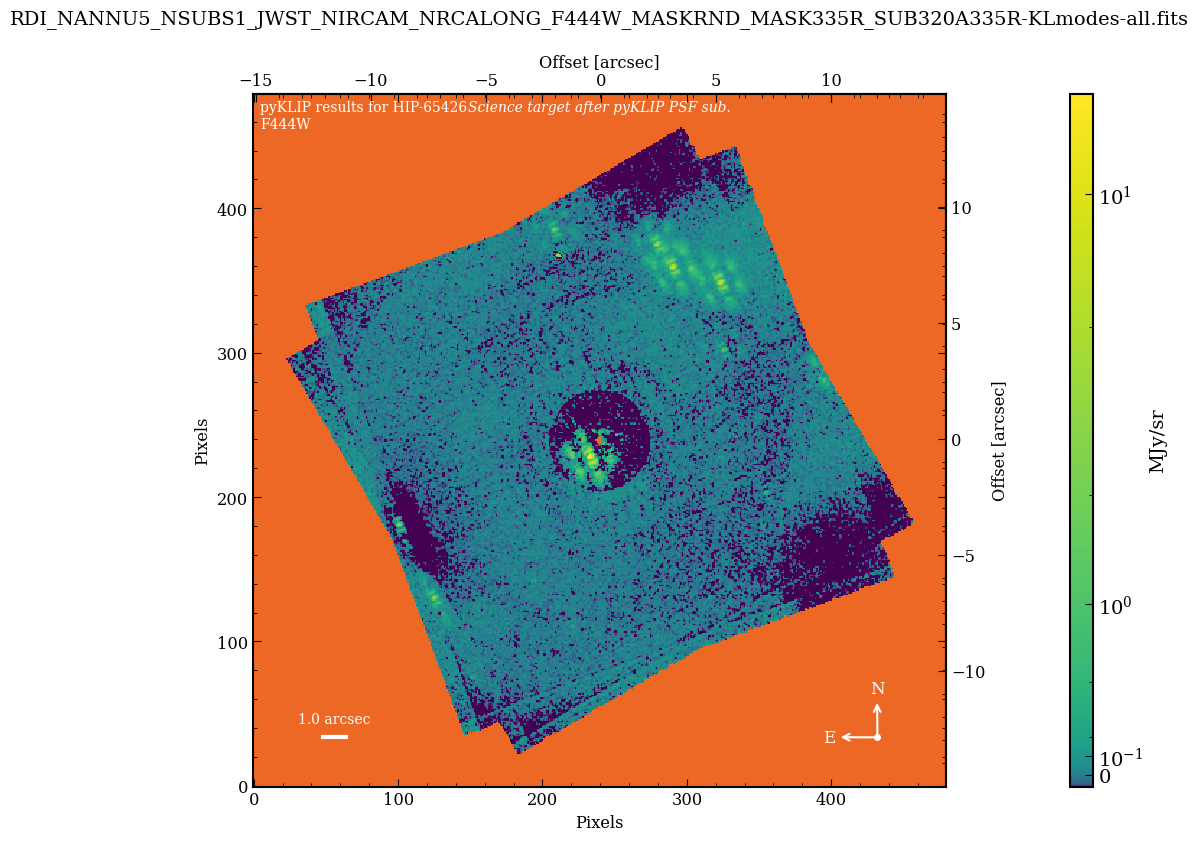
PSF Subtraction: option using classical PSF subtraction
In some cases, simple classical PSF subtraction is a robust and effective approach.
We also demonstrate here the save_rolls
parameter, which causes the PSF-subtracted versions of each individual science roll to also be saved, in addition to the roll-combined final product.
TODO: debug the following…
[36]:
spaceKLIP.classpsfsubpipeline.run_obs(database,
subdir='classical',
kwargs = {'save_rolls': True})
[spaceKLIP.classpsfsubpipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F250M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/numpy/lib/nanfunctions.py:1217: RuntimeWarning: All-NaN slice encountered
[py.warnings:WARNING] return function_base._ureduce(a, func=_nanmedian, keepdims=keepdims,
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T01:54:23.183' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:04:41.088' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:14:58.993' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.395270 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.218416 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706457085.839 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.classpsfsubpipeline:INFO] --> Average best fit scaling factor (dpos1) = 1.00
[spaceKLIP.classpsfsubpipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F300M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/classpsfsubpipeline.py:194: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03106_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = pyfits.HDUList([hdu0])
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:17:11.949' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:27:29.854' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:37:47.759' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.390994 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.213823 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706416105.011 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.classpsfsubpipeline:INFO] --> Average best fit scaling factor (dpos1) = 1.00
[spaceKLIP.classpsfsubpipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F356W_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/classpsfsubpipeline.py:194: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03107_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = pyfits.HDUList([hdu0])
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:52:36.861' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:57:45.813' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:02:54.766' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.385314 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.207724 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706361731.693 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.classpsfsubpipeline:INFO] --> Average best fit scaling factor (dpos1) = 1.00
[spaceKLIP.classpsfsubpipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F410M_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/classpsfsubpipeline.py:194: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03109_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = pyfits.HDUList([hdu0])
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T02:40:02.814' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T02:45:11.766' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T02:50:20.719' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.387673 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.210257 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706384309.811 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.classpsfsubpipeline:INFO] --> Average best fit scaling factor (dpos1) = 1.00
[spaceKLIP.classpsfsubpipeline:INFO] --> Concatenation JWST_NIRCAM_NRCALONG_F444W_MASKRND_MASK335R_SUB320A335R
[py.warnings:WARNING] /Users/mperrin/Dropbox (Personal)/Documents/software/git/spaceKLIP/spaceKLIP/classpsfsubpipeline.py:194: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_03108_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] hdul = pyfits.HDUList([hdu0])
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'datfix' made the change 'Set DATE-BEG to '2022-07-30T03:05:08.796' from MJD-BEG.
[py.warnings:WARNING] Set DATE-AVG to '2022-07-30T03:10:17.748' from MJD-AVG.
[py.warnings:WARNING] Set DATE-END to '2022-07-30T03:15:26.701' from MJD-END'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[py.warnings:WARNING] /Users/mperrin/miniconda3/envs/stenv-py3.11-2023.06.08/lib/python3.11/site-packages/astropy/wcs/wcs.py:819: FITSFixedWarning: 'obsfix' made the change 'Set OBSGEO-L to -67.382959 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-B to -35.205196 from OBSGEO-[XYZ].
[py.warnings:WARNING] Set OBSGEO-H to 1706339216.087 from OBSGEO-[XYZ]'.
[py.warnings:WARNING] warnings.warn(
[py.warnings:WARNING]
[spaceKLIP.classpsfsubpipeline:INFO] --> Average best fit scaling factor (dpos1) = 1.00
[py.warnings:WARNING] /var/folders/wj/p62fp78j0z1b4xyr2yql1prw00042w/T/ipykernel_27683/799678275.py:1: ResourceWarning: unclosed file <_io.BufferedReader name='data_nircam_hd65426/padded/jw01386002001_0310a_00001_nrcalong_calints_psfmask.fits'>
[py.warnings:WARNING] spaceKLIP.classpsfsubpipeline.run_obs(database,
[py.warnings:WARNING]
[37]:
!open .
IOStream.flush timed out
IOStream.flush timed out
IOStream.flush timed out
[ ]: